Micronaut OpenAPI
As we all know Spring there is now a lightweigth and claimed more efficient framework called Micronaut. The key difference is it instruments the code AOT (ahead of time) ensuring less memory footprint (less caching, less dymanic proxy to be created) and so a quicker start time.
Cli Installation
Micronaut has a CLI to scaffold your application
- install micronaut-cli using a package manager as per Micronaut documentation
- ensure
$MICRONAUT_CLI_HOME/bin
toPATH
; it will be referred asmn
- check installation
mn --version
Windows
Using Chocolatey
choco install micronaut
Ubuntu
With SDKMAN
sdk install micronaut
Sample Application
You can use Mirconaut CLI to initiate your application
> mn create-app hello-world --build maven --features openapi
The project is scaffolded and the main class is generated
public class Application {
public static void main(String[] args) {
Micronaut.run(Application.class, args);
}
}
You can start adding a Controller to spawn a server side application
@Controller("/service")
public class ServiceController {
@Post("execute")
@Status(HttpStatus.CREATED)
public Single<Result> execute(@Body Request request) {
Result result = new Result();
result.setValue(request.getValue() * 2);
return Single.just(result);
}
}
To compile or build simply run mvn compile
or mvn install
, or use mn
mvnw mn:run
Maven Dependencies
In in your maven pom.xml
- add compile dependencies
<dependency>
<groupId>javax.annotation</groupId>
<artifactId>javax.annotation-api</artifactId>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>io.swagger.core.v3</groupId>
<artifactId>swagger-annotations</artifactId>
<scope>compile</scope>
</dependency>
- configure annotation processor
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<annotationProcessorPaths combine.children="append">
<path>
<groupId>io.micronaut.openapi</groupId>
<artifactId>micronaut-openapi</artifactId>
<version>${micronaut.openapi.version}</version>
</path>
</annotationProcessorPaths>
<compilerArgs>
<arg>-Amicronaut.processing.group=fr.kdefombelle.app</arg>
<arg>-Amicronaut.processing.module=app-service</arg>
</compilerArgs>
</configuration>
</plugin>
Swagger
Reference documentation is Micronaut Open API
In your application properties point swagger static resources
micronaut:
application:
name: sample
router:
static-resources:
swagger:
paths: classpath:META-INF/swagger
mapping: /swagger/**
swagger-ui:
paths: classpath:META-INF/swagger/views/swagger-ui
mapping: /swagger-ui/**
Document the API
@Operation(
summary = "Double value",
description = "Simple webservice which doubles a value provided by the requester")
@ApiResponse(
responseCode = "201",
description = "Response object",
content = @Content(mediaType = "application/json",
schema = @Schema(type = "Response")
)
)
@ApiResponse(
responseCode = "400",
description = "Response object",
content = @Content(
mediaType = "application/json",
schema = @Schema(type = "Response")
)
)
@Post("execute")
@Status(HttpStatus.CREATED)
public Single<Result> execute(@Body Request request) {
Result result = new Result();
result.setValue(request.getValue() * 2);
return Single.just(result);
}
Enable Swagger
Reference documentation is Micronaut Open API
Create an openapi.properties
file at the root of your project containing
swagger-ui.enabled=true
In your application properties point swagger static resources, e.g.: in application.yml
micronaut:
application:
name: sample
router:
static-resources:
swagger:
paths: classpath:META-INF/swagger
mapping: /swagger/**
swagger-ui:
paths: classpath:META-INF/swagger/views/swagger-ui
mapping: /swagger-ui/**
Visualise
To access swagger you can run your app and go to URL http://localhost:8080/swagger-ui
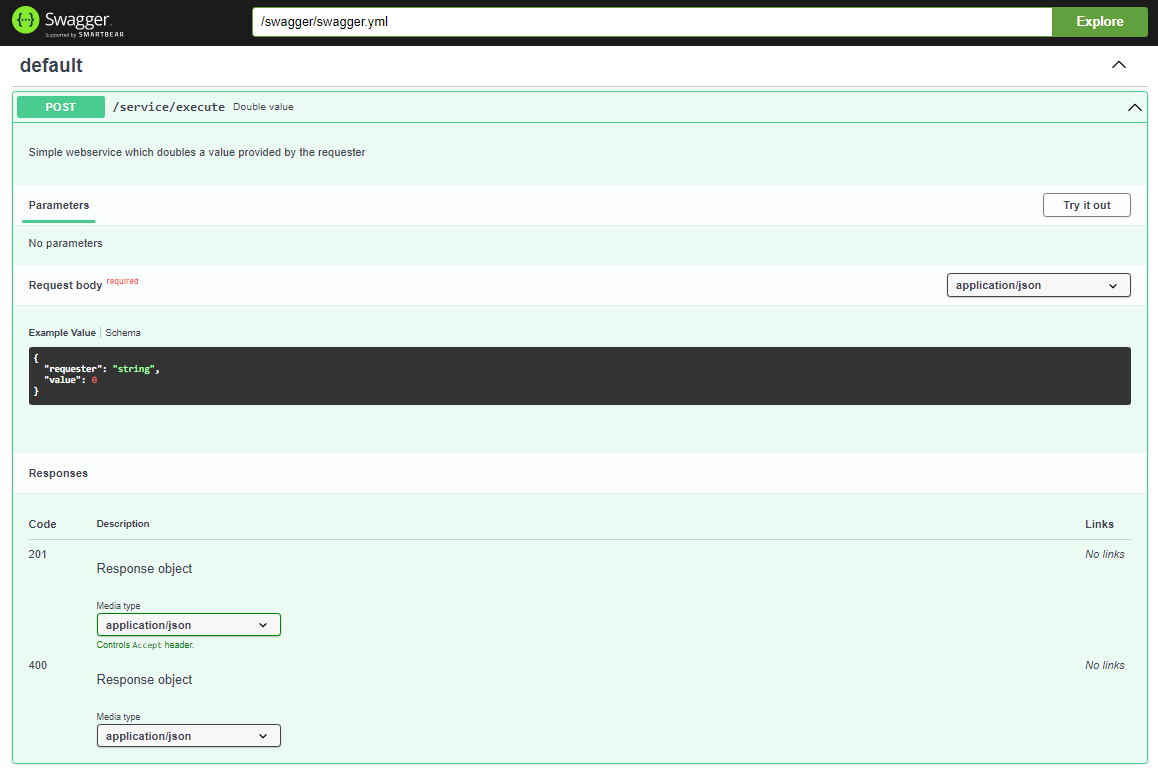
Enjoy Reading This Article?
Here are some more articles you might like to read next: